Table of Contents
- Python GUI app for Calendar | Aman Kharwal
- Create a GUI Calendar using PyQt5 in Python - Javatpoint
- How to create GUI calendar using python - YouTube
- Python GUI Project Tutorial Part 03: Converting UI File to Python ...
- Pyside2 GUI | Making Center The Window | Qt For Python | Python GUI ...
- | How to Create a GUI Calendar using python( 8 lines) | | AK | - YouTube
- Python 3 - Modern GUI / Flat GUI / Layout / Interface - PySide2 or ...
- How To Build A Calendar In Python Using Tkinter Build - vrogue.co
- Python Gui How To Create Aboutbox In Pyside2 Codeloop - vrogue.co
- Python GUI Working With GridLayout Of Pyside2 Qt For Python - YouTube



What is PySide2?
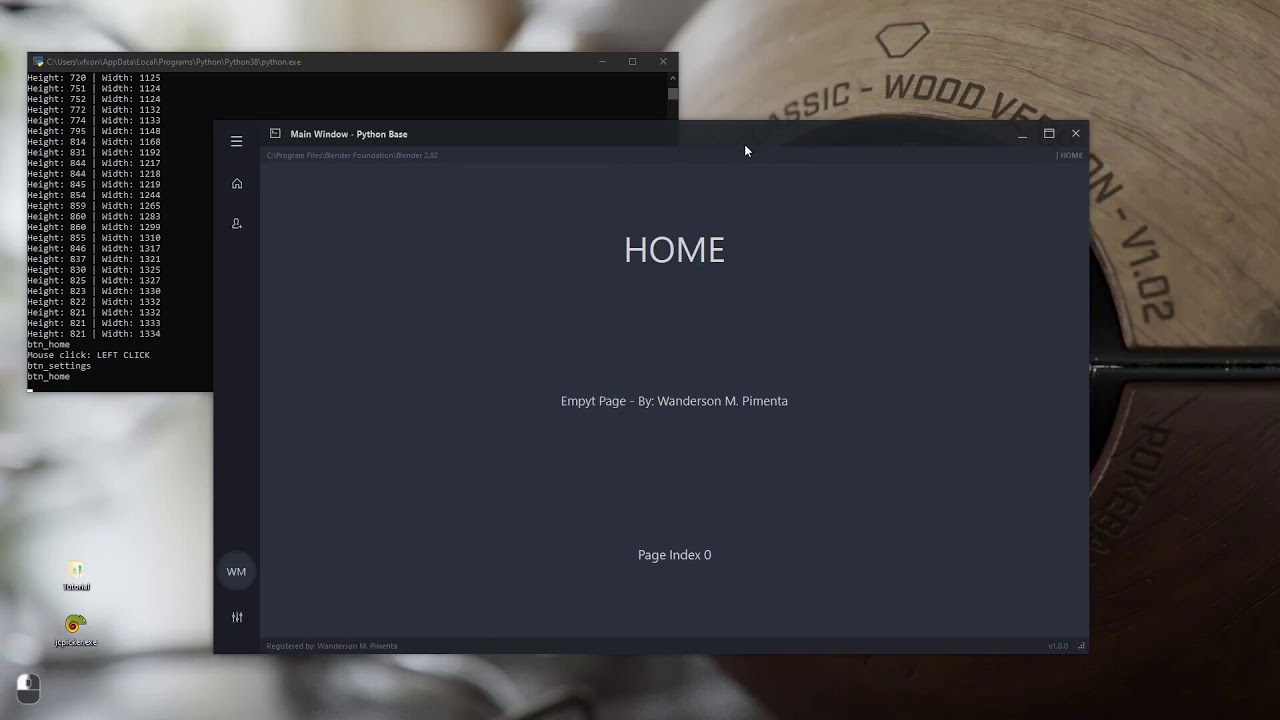
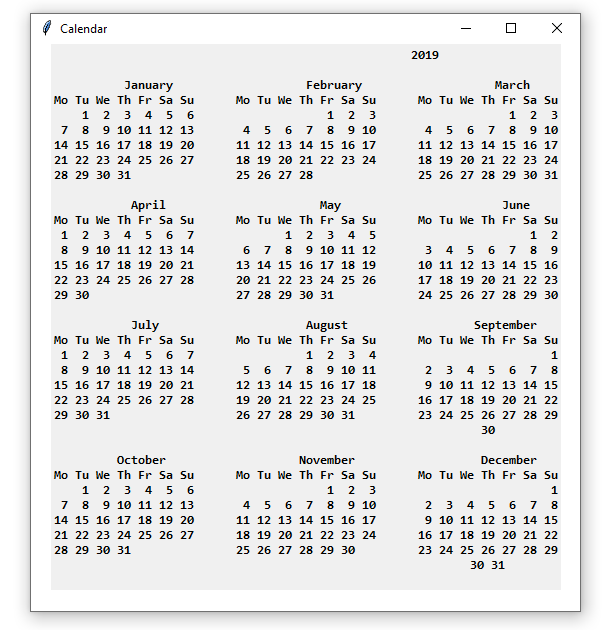
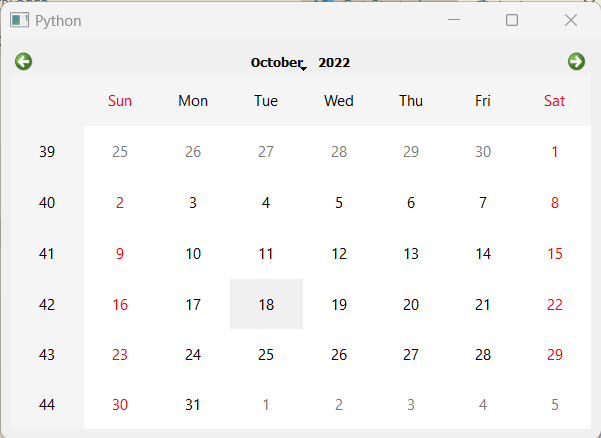
Installing PySide2
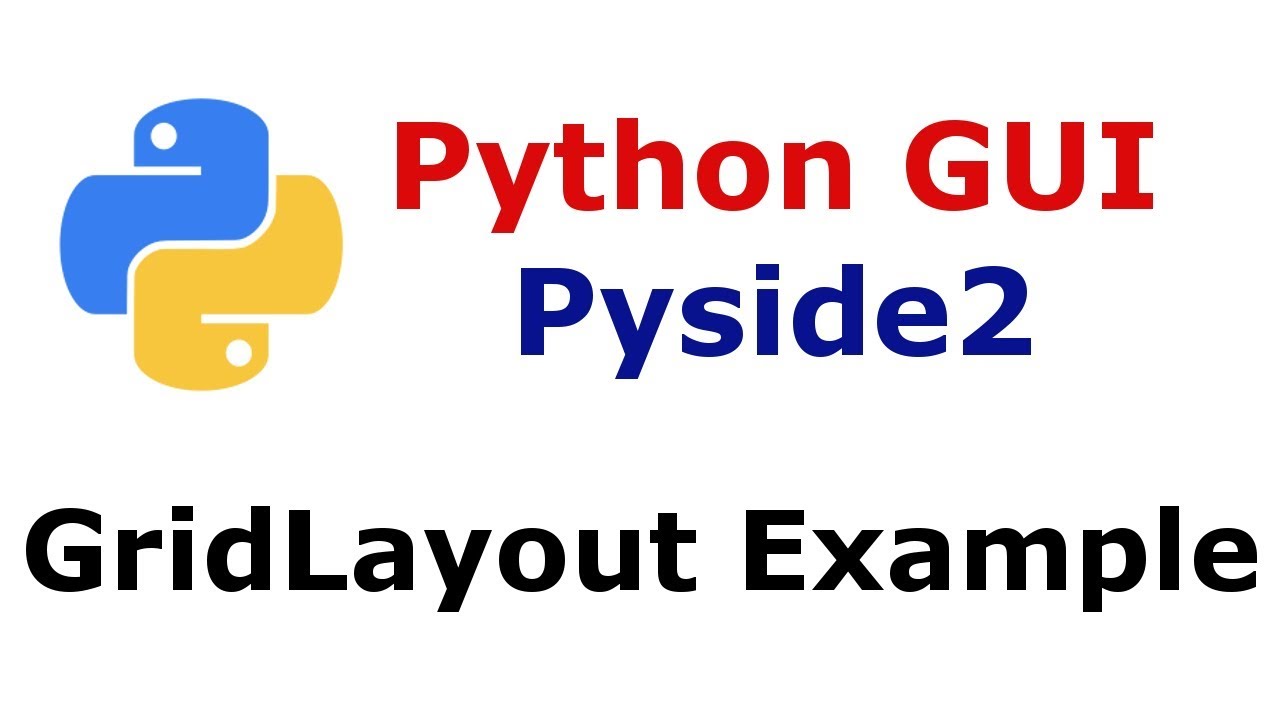
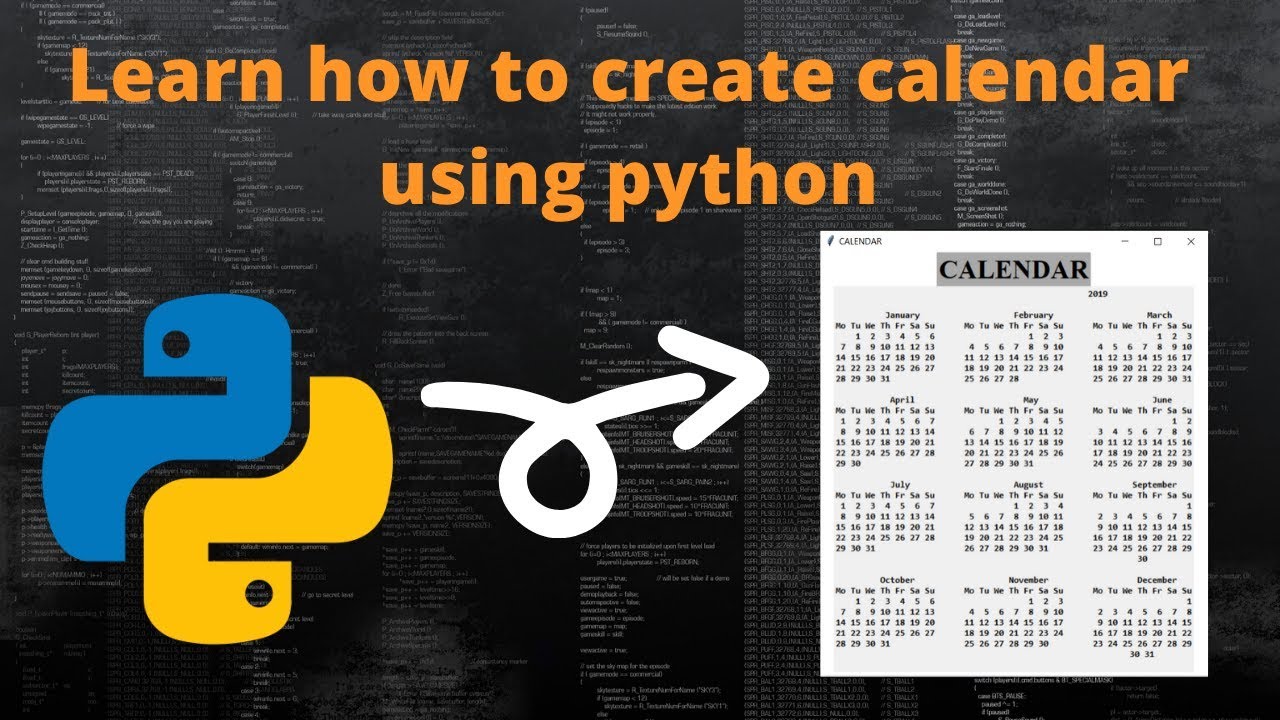
Creating Your First GUI Application
Now that you have PySide2 installed, let's create a simple "Hello World" GUI application. Here's the code: ```python import sys from PySide2.QtWidgets import QApplication, QLabel if __name__ == "__main__": app = QApplication(sys.argv) label = QLabel("Hello, World!") label.show() sys.exit(app.exec_()) ``` Let's break down what's happening in this code: We import the necessary modules, including `sys` and `QApplication` and `QLabel` from PySide2. We create a `QApplication` instance, which is the main entry point for any Qt-based GUI application. We create a `QLabel` instance, which is a simple widget that displays text. We set the text of the label to "Hello, World!" and show it on the screen. Finally, we start the application's event loop with `app.exec_()` and exit the application when it's closed.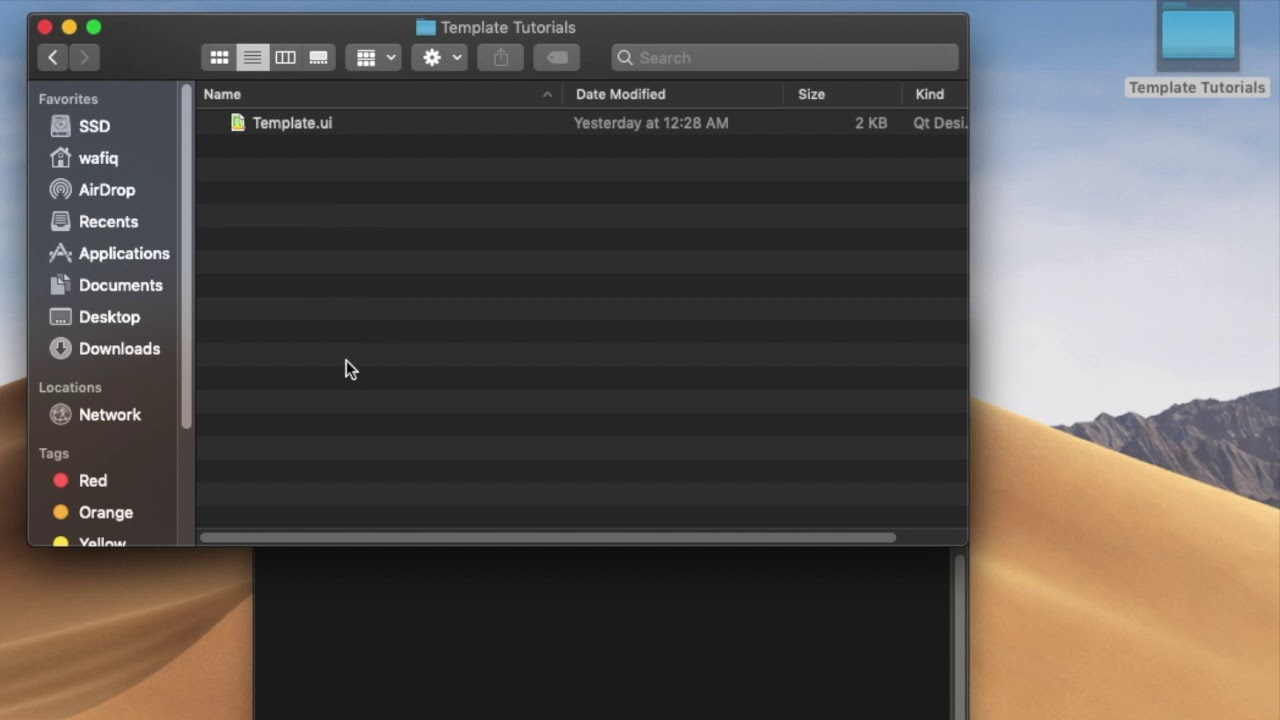
Running Your GUI Application
To run your GUI application, save the code above to a file (e.g., `hello_world.py`) and run it with Python: ```bash python hello_world.py ``` You should see a simple window with the text "Hello, World!". In this article, we've shown you how to create a simple GUI application with PySide2, a powerful and popular library for building graphical user interfaces with Python. With its comprehensive set of libraries and tools, PySide2 makes it easy to build complex GUI applications, and its large community and extensive documentation make it a great choice for developers of all levels. Whether you're building a simple "Hello World" app or a complex data-driven GUI application, PySide2 is a great choice. By following the steps outlined in this article, you can create your own GUI applications with PySide2 and start building the next generation of graphical user interfaces. So why wait? Start building your GUI application today and see what you can create!Keyword: PySide2, Python GUI, GUI Application, Qt, PyQt, PySide